Web page capturing API from Fireshot
Introduction
By using this API and Javascript, you can embed web page capturing functions into your website. For example, this can be a button or just an event that launches FireShot and opens the editor with the captured web page. You can think of the other ways of using FireShot, such as:
- Saving screenshots to disk
- Uploading to web
- Copying to clipboard
- E-mailing
- Exporting to external editor
- Printing
The embedding is quick and simple.
Security notice
Because of the security concerns, the FireShot API should be explicitly enabled in the FireShot options.
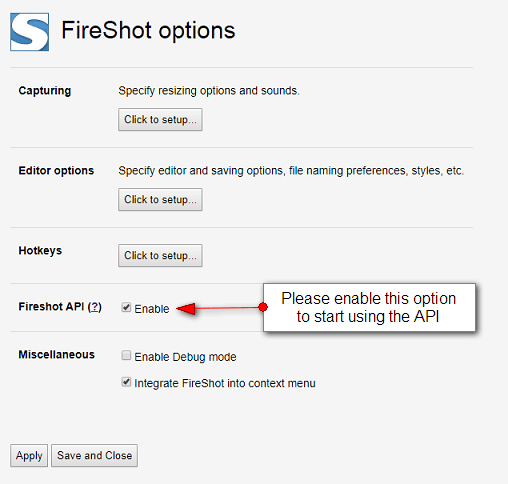
Prerequisites
To be capable of taking web page captures using Javascript, the following should be installed:
- Google Chrome (or any Chromium-based browser supporting FireShot), Opera or Mozilla Firefox.
- FireShot extension (v.0.98.23 and higher) Pro version or lite (https://getfireshot.com)
Demo #1: Implementing the addon check
Source code:
<html> <head> <script type="text/javascript" src="fsapi.js"></script> <script type="text/javascript"> function checkFSAPI() { var element = document.getElementById("spnPluginStatus"); if (typeof (FireShotAPI) != "undefined" && FireShotAPI.isAvailable()) element.innerHTML = "<b>Installed and ready!</b>"; else element.innerHTML = "<b>Not installed</b>, please see the " + "<a href='https://getfireshot.com/api-required.php'>troubleshooting page</a>"; } </script> </head> <body> <p>FireShot addon status: <span id="spnPluginStatus">unknown, press "Check Status" button</span> <br> <br> <input type="button" onClick="checkFSAPI()" value="Check Status" /> </p> </body> </html>
Demo #2: Using the addon to capture web pages
This demo shows all the ways that FireShot may be used.
To use the full functionality (such as editing, saving, copying, etc), please enable the Advanced capturing features in FireShot.
Please note, that if you're using the Lite version, the captured page will be opened in a new tab, no matter what option you choose.
Source code:
<html> <head> <script type="text/javascript" src="fsapi.js" onerror="alert('Error: failed to load ' + this.src)"> </script> <script> // Set this to *false* to avoid addon auto-installation if missed. FireShotAPI.AutoInstall = true; function screenshotToBase64(mode) { FireShotAPI.base64EncodePage(mode, undefined, function (data) { var w = window.open('', 'View captured image', 'width=800,height=600,toolbar=1,scrollbars=1'); w.document.write('<title>Preview base64 image</title>'); w.document.write('<p><b>This is a captured image embedded into HTML by JavaScript.</b></p>'); var img = w.document.createElement("IMG"); img.style = "max-width:100%; height:auto"; img.src = data; w.document.body.appendChild(img); }); } function checkAvailability() { // We need to wait a little bit for the FS API initialization. setTimeout(function() { FireShotAPI.checkAvailability(); }, 1000); } document.addEventListener("DOMContentLoaded", checkAvailability); </script> </head> <body> <div align="center"> <form> <p>Capture entire webpage and:</p> <p><strong>Capture entire webpage and:</strong></p> <input type="button" onClick="FireShotAPI.editPage(true)" value="Edit"> <input type="button" onClick="FireShotAPI.savePage(true)" value="Save"> <input type="button" onClick="FireShotAPI.copyPage(true)" value="Copy"> <input type="button" onClick="FireShotAPI.emailPage(true)" value="E-Mail"> <input type="button" onClick="FireShotAPI.exportPage(true)" value="Export"> <input type="button" onClick="FireShotAPI.uploadPage(true)" value="Upload"> <input type="button" onClick="FireShotAPI.printPage(true)" value="Print"> <input type="button" onClick="screenshotToBase64(true)" value="BASE64"> <br><br> <p><strong>Capture visible part and:</strong></p> <input type="button" onClick="FireShotAPI.editPage(false)" value="Edit"> <input type="button" onClick="FireShotAPI.savePage(false)" value="Save"> <input type="button" onClick="FireShotAPI.copyPage(false)" value="Copy"> <input type="button" onClick="FireShotAPI.emailPage(false)" value="E-Mail"> <input type="button" onClick="FireShotAPI.exportPage(false)" value="Export"> <input type="button" onClick="FireShotAPI.uploadPage(false)" value="Upload"> <input type="button" onClick="FireShotAPI.printPage(false)" value="Print"> <input type="button" onClick="screenshotToBase64(false)" value="BASE64"> </form> </div> </body> </html>
Demo #3: Live FireShot application
This demo shows how to check whether FireShot is installed and how to capture the current web page and send it via E-mail.
Source code:
<html> <head> <script type="text/javascript" src="fsapi.js" onerror="alert('Error: failed to load ' + this.src)"> </script> <script> // Set this to *false* to avoid addon auto-installation if missed. FireShotAPI.AutoInstall = true; </script> </head> <body> <div align="center"> <form> <p>See errors at the page?</p> <input type="button" onClick="FireShotAPI.emailPage(true)" value="Click to email this page how you see it."> </form> </div> </body> </html>
Links
Download the API library and demos (4 KB)
Feature requests / Bug reports